Build with SFPY - Part 1
On SFPY, anyone can integrate free decentralized payments in a traditional commerce setting using our APIs built on top of Ethereum and our payments protocol. This tutorial series will focus on developers looking to add crypto payments acceptance to their websites through an easy to use REST API.
The following topics will be covered but will be broken up into parts for brevity:
- Installing the
go-sfpy
library - Initialize the client.
- Exposing an endpoint to create a new order
- Redirecting the customer to complete the payment.
I hope you find this useful and interesting enough to follow along. Future tutorials will cover how to integrate payments through smart contracts to showcase the flexibility of SFPY.
If you want to skip right ahead to a repository I have published you can head on over here
#
Part 1 - Installing the go-sfpy libraryAssuming you have a go service that you're working on, you can easily install our native SDK. Installation instructions are available here but I'll repeat them for convenience.
In your terminal, cd
to the root of your service and make sure it is using Go Modules. If not, you can convert your service into a module by typing
Then, reference go-sfpy in a Go file with import
:
Alternatively, you can also explicitly go get the package into a project:
#
Part 2 - Initialize the sfpy clientTo use the SFPY client to make API requests, initialize it like so from within your service
NewClient
accepts two arguments:
apikey
: This is your SFPY Api key that is needed to authenticate all requests to the API. You can find this key on your dashboard.secretkey
: This is your webhook shared secret key used to validate whether incoming signatures from webhooks are valid.
Its almost always better to initialize this as a singleton when your service is starting up and pass it as an argument to your request handlers. For instance if you have a controller that handles incoming requests to create new, unpaid orders, you can pass the client as an argument like so:
#
Service handler struct#
Service instantiationBefore initializing this service, you would initialize the sfpy client and pass that in as an argument like so:
#
Part 3 - Exposing an endpoint to create a new orderIn a traditional commerce setting, customers add items to a cart, or make a purchase selection with the intention of paying for the good or service. At this point, developers are usually familiar with integrating something like Stripe or PayPal which handles collecting payment information on an externally hosted page, redirects the customer back to the store after a successful payment and notifies the merchant of a successful payment through a webhook so that the system can reconcile the order and mark it paid. This is the exact flow we will cover below.
Assuming you have an API endpoint hosted on your server to initialize an order, you will want to create a payment request on SFPY with details like total amount to charge, any optional discount or any optional tax to add. An overly simplified API contract is presented below:
Decode an incoming HTTP request into this struct
Handle the business logic and API call to SFPY in your service (defined above)
#
Step 4 - Complete the paymentOnce you've successfully performed the API request, you can return the generated payment link back to the front end. With javascript, after parsing the response, you can redirect the customer to SFPY to complete the payment as follows:
Hopefully, if everything goes right, your customer will be redirected to SFPY to complete their payment using one of the 5 currently supported currencies:
- ETH
- USDC
- DAI
- TETHER
- WBTC
#
Customer payments experienceAs an example, this is what your customer would experience.
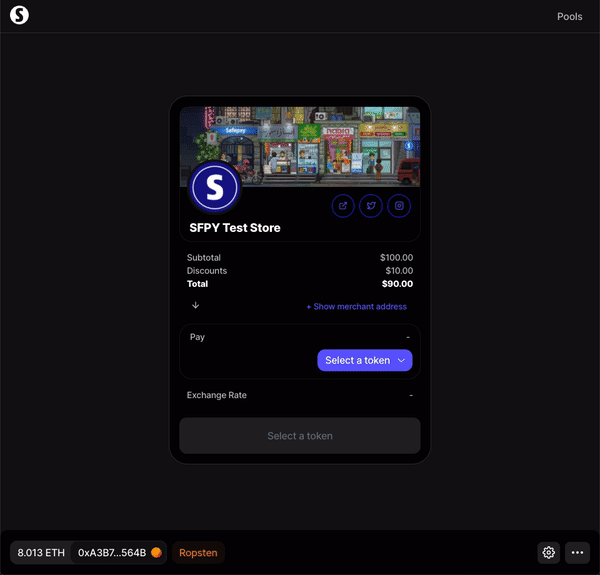
#
ConclusionThank you for following along! In the next episode, we'll learn how to handle incoming webhooks in order to programmatically mark the order as PAID
and save payment details to your database for future reference.
In the meantime, if you have any questions, please join us on our Discord Server. We're more than happy to help troubleshoot any problems you face.